Polymorphism
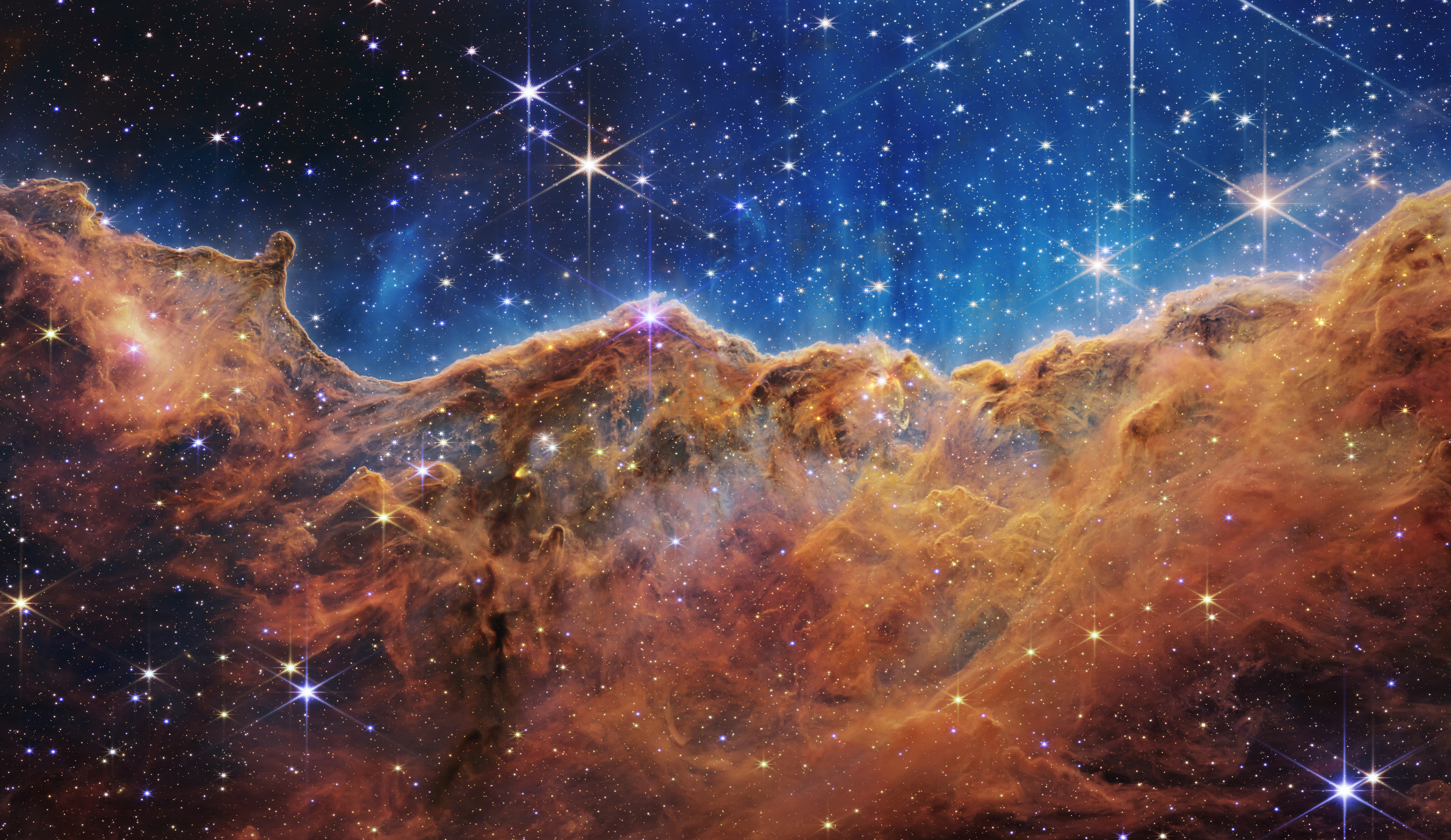
Polymorphism is a programming concept that refers to the ability of a single piece of code to work with multiple data types or objects. Polymorphism can be achieved through inheritance, where a child class inherits from a parent class and can be used interchangeably with the parent class, or through the use of interfaces, where a class can implement one or more interfaces and must provide an implementation for each method defined in the interface.
Polymorphism is a powerful technique that can make your code more flexible and adaptable, because it allows you to write code that can work with multiple data types or objects without having to know the specific details of each type or object.
Here is an example of polymorphism in Python using inheritance:
# Define a parent class "Shape"
class Shape:
def area(self):
pass # This method will be implemented by child classes
# Define a child class "Rectangle" that inherits from "Shape"
class Rectangle(Shape):
def __init__(self, width, height):
self.width = width
self.height = height
def area(self):
return self.width * self.height
# Define a child class "Circle" that inherits from "Shape"
class Circle(Shape):
def __init__(self, radius):
self.radius = radius
def area(self):
return 3.14 * self.radius * self.radius
# Create a list of "Shape" objects
shapes = [Rectangle(10, 20), Circle(5)]
# Calculate the area of each shape
for shape in shapes:
print(shape.area())
In this example, the Rectangle and Circle classes both inherit from the Shape class and implement the area method. This allows you to write code that works with any class that inherits from the Shape class, without having to know the specific details of each class.
You should use polymorphism when you want to write code that can work with multiple data types or objects without having to know the specific details of each type or object.
Polymorphism is a powerful technique that can make your code more flexible and adaptable, because it allows you to write code that can be used with a wide range of data types or objects. This can be particularly useful in situations where you don't know in advance which data types or objects your code will need to work with, or when you want to add new types or objects to your system in the future.
Here are a few examples of when you might use polymorphism:
-
You want to write a function that can work with multiple data types, such as integers, floating-point numbers, and strings.
-
You want to create a class hierarchy, where a child class inherits from a parent class and can be used interchangeably with the parent class.
-
You want to create a flexible and adaptable system that can be extended with new classes and functionality in the future.
Overall, polymorphism is a useful tool for building modular and flexible object-oriented systems, and it can help you to write code that is more reusable, maintainable, and adaptable. However, it is important to use polymorphism appropriately and to consider whether it is the most appropriate solution for your problem.