Classes
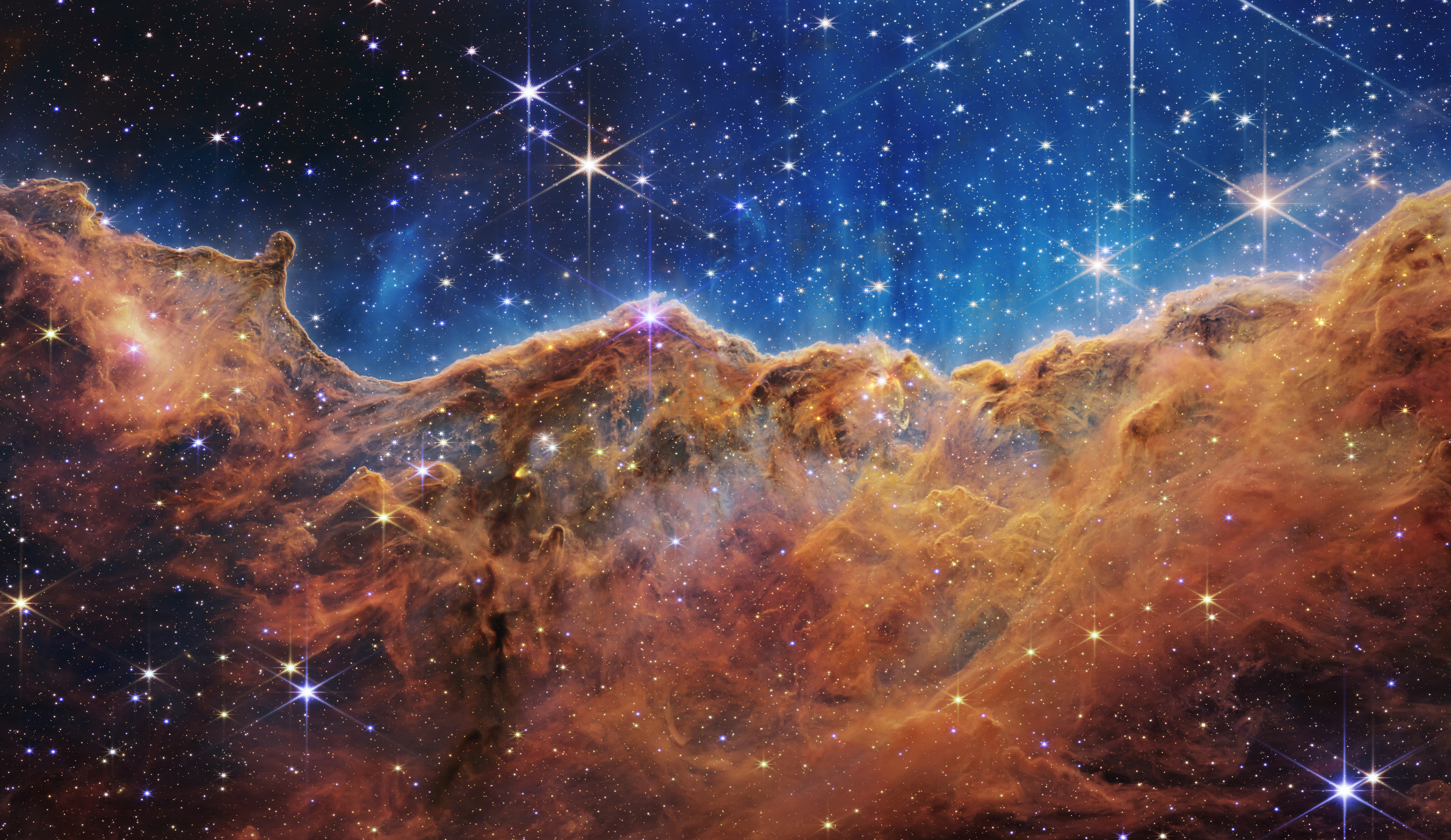
A class is a blueprint for creating objects (a particular data structure), providing initial values for state (member variables or attributes), and implementations of behavior (member functions or methods). In object-oriented programming, a class defines a type of object, but it is not an object itself. Instead, an object is an instance of a class.
Classes are a fundamental concept in many programming languages such as C++, Java, Python, and C#. They provide a way to organize and structure code, making it more modular and reusable. Classes allow for the encapsulation of data and behavior, which helps to hide the implementation details of an object and protect its internal state from being modified by other parts of the code. This is known as data hiding or information hiding.
In most object-oriented languages, classes have two main components: fields (attributes or member variables) and methods (member functions). Fields are used to store the state of an object, while methods are used to define the behavior of the object. For example, a class for a Car
object might have fields for the make, model, and current speed, and methods for accelerating and braking.
An example of a class in Python is as follows:
class Car:
def __init__(self, make, model):
self.make = make
self.model = model
self.speed = 0
def accelerate(self, amount):
self.speed += amount
def brake(self):
self.speed = 0
def get_speed(self):
return self.speed
my_car = Car("Toyota", "Camry")
print(my_car.make) # Output: Toyota
print(my_car.model) # Output: Camry
print(my_car.get_speed()) # Output: 0
my_car.accelerate(20)
print(my_car.get_speed()) # Output: 20
my_car.brake()
print(my_car.get_speed()) # Output: 0
In this example, we have defined a class called Car
. The class has three fields: make
, model
, and speed
. The constructor method __init__
is used to initialize the fields when a new object of the class is created. The class also has three methods: accelerate
, brake
, and get_speed
. The accelerate
method increases the speed of the car by a certain amount, the brake
method sets the speed to 0, and the get_speed
method returns the current speed of the car.
We then create an object of the Car
class called my_car
and initialize it with the make "Toyota" and the model "Camry". We can access the fields of the class using the dot notation (e.g., my_car.make
) and we can call the methods of the class using the same notation (e.g., my_car.accelerate(20)
).
Classes can also be organized into hierarchies, where a class can inherit from another class. This is known as inheritance, and it allows for the reuse of code and behavior. Child classes inherit the fields and methods of the parent class and can also add new fields and methods or override the ones inherited from the parent class. This is called polymorphism, and it allows for the creation of objects with different behavior but the same interface.
class ElectricCar(Car):
def __init__(self, make, model, battery_capacity):
super().__init__(make, model)
self.battery_capacity = battery_capacity
def get_battery_capacity(self):
return self.battery_capacity
my_electric_car = ElectricCar(`Tesla`, `Model S`, 100)
print(my_electric_car.make) # Output: Tesla
print(my_electric_car.model) # Output: Model S
print(my_electric_car.get_battery_capacity()) # Output: 100
In this example, we have defined a new class called ElectricCar
which inherits from the Car
class. The ElectricCar
class has an additional field battery_capacity
and an additional method get_battery_capacity()
. By inheriting from the Car
class, the ElectricCar
class automatically has the make
, model
, speed
fields, and accelerate
, brake
, get_speed
methods from the Car
class, and we can use them as is.
In addition to inheritance, classes can also be related to each other using composition, which allows an object to contain other objects. Composition allows for the creation of complex objects by combining simpler objects.
class Engine:
def __init__(self, horsepower):
self.horsepower = horsepower
def get_horsepower(self):
return self.horsepower
class CompositionCar:
def __init__(self, make, model):
self.make = make
self.model = model
self.engine = Engine(200)
def get
In the composition example, we have defined two classes: Engine
and CompositionCar
. The Engine
class has a field horsepower
and a method get_horsepower()
that returns the horsepower of the engine. The CompositionCar
class has fields make
and model
, and an additional field engine
which is an instance of the Engine
class.
The constructor method __init__
of the CompositionCar
class initializes the make, model fields and create an instance of the Engine
class with a horsepower of 200. This is composition, where the class CompositionCar
is composed of an object of the class Engine
The CompositionCar class doesn't inherit from Engine, but it has an object of Engine as a field, it uses the functionality of Engine class but doesn't inherit it. This allows for more flexibility in creating complex objects, for example, a car can have multiple engines, and we can easily switch them by just changing the instance of the engine object.
In short, composition allows an object to contain other objects, it allows for the creation of complex objects by combining simpler objects, and it allows for the flexibility of changing the contained objects if needed.
In summary, classes are a fundamental concept in object-oriented programming that provide a blueprint for creating objects, encapsulating data and behavior, and organizing and structuring code. Classes have fields and methods and can be organized into hierarchies using inheritance and composition.