Interface
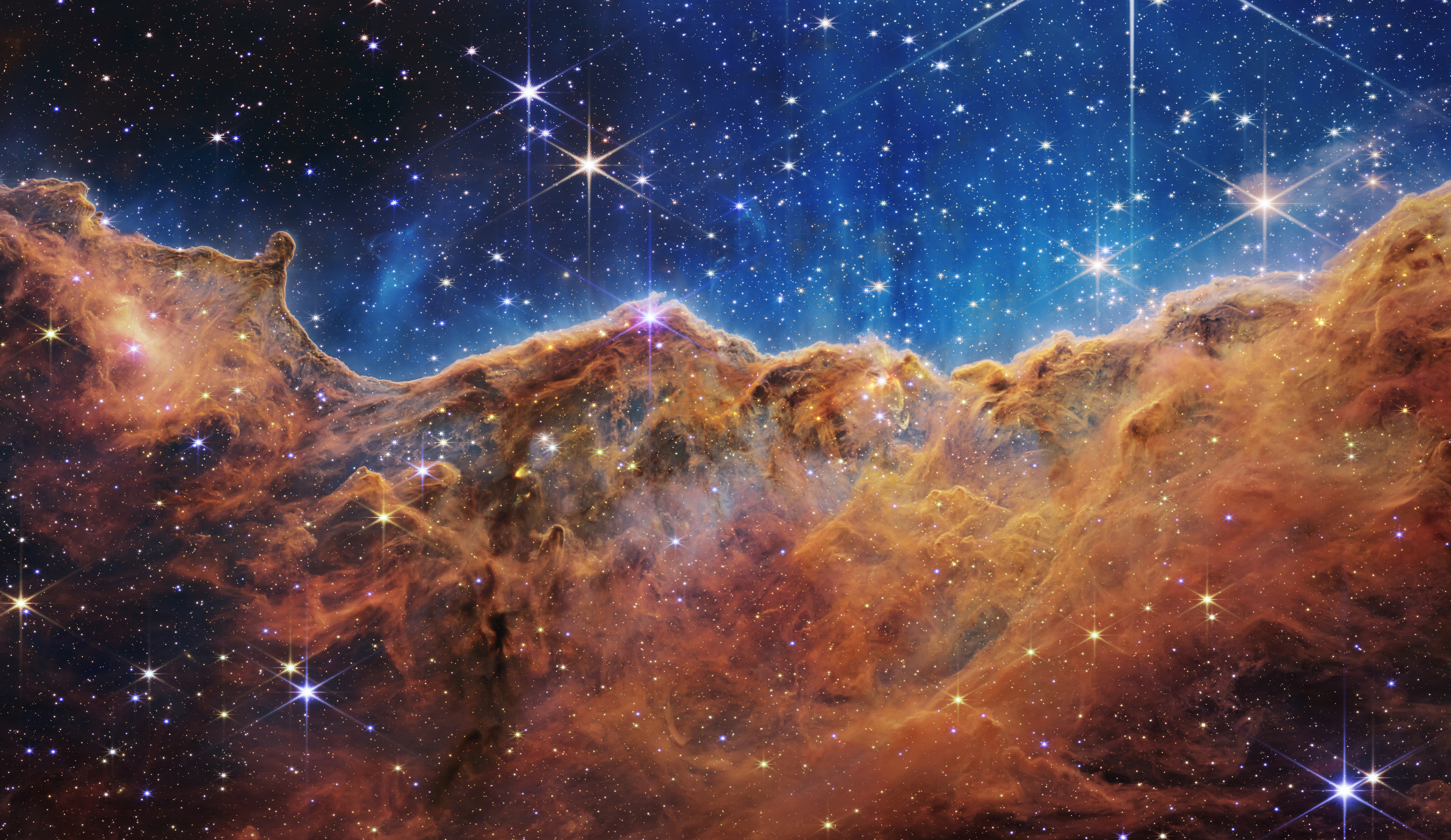
An interface is a way to specify a set of related methods that a class must implement, without actually specifying the implementation of those methods. Interfaces are used to define contracts in which a class agrees to implement a certain set of methods, but leaves the actual implementation up to the class.
Interfaces are used to define a common set of methods that can be used to interact with a group of related classes. This allows you to write code that is more flexible and adaptable, because you can write code that works with any class that implements the interface, rather than being tied to a specific class.
Here's an example of how to use interfaces in Python:
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def make_sound(self):
pass
class Dog(Animal):
def make_sound(self):
print("Woof!")
class Cat(Animal):
def make_sound(self):
print("Meow!")
dog = Dog()
cat = Cat()
dog.make_sound() # Output: "Woof!"
cat.make_sound() # Output: "Meow!"
In this example, we have an Animal
interface that defines an abstractmethod
called make_sound
. This means that any class that implements the Animal
interface must have a make_sound
method.
We have two classes, Dog
and Cat
, that both implement the Animal
interface. Each class provides its own implementation of the make_sound
method.
We can then create instances of the Dog
and Cat
classes and call the make_sound
method on them. The correct implementation of the method will be called based on the type of object it is called on.
Interfaces are a useful technique for creating a hierarchy of classes, where a class can implement one or more interfaces and must provide an implementation for each method defined in the interface. This allows you to write code that works with any class that implements the interface, without having to know the specific details of the class.
Here are a few examples of when you might use interfaces:
-
You want to write code that can work with multiple implementations of the same concept, such as different shapes or different data storage devices.
-
You want to define a common set of methods that can be used to interact with a group of related classes, such as a set of database access classes.
-
You want to create a flexible and adaptable system that can be extended with new classes and functionality in the future.
Overall, interfaces are a useful tool for building modular and flexible object-oriented systems, and they can help you to write code that is more reusable, maintainable, and adaptable.