Data types
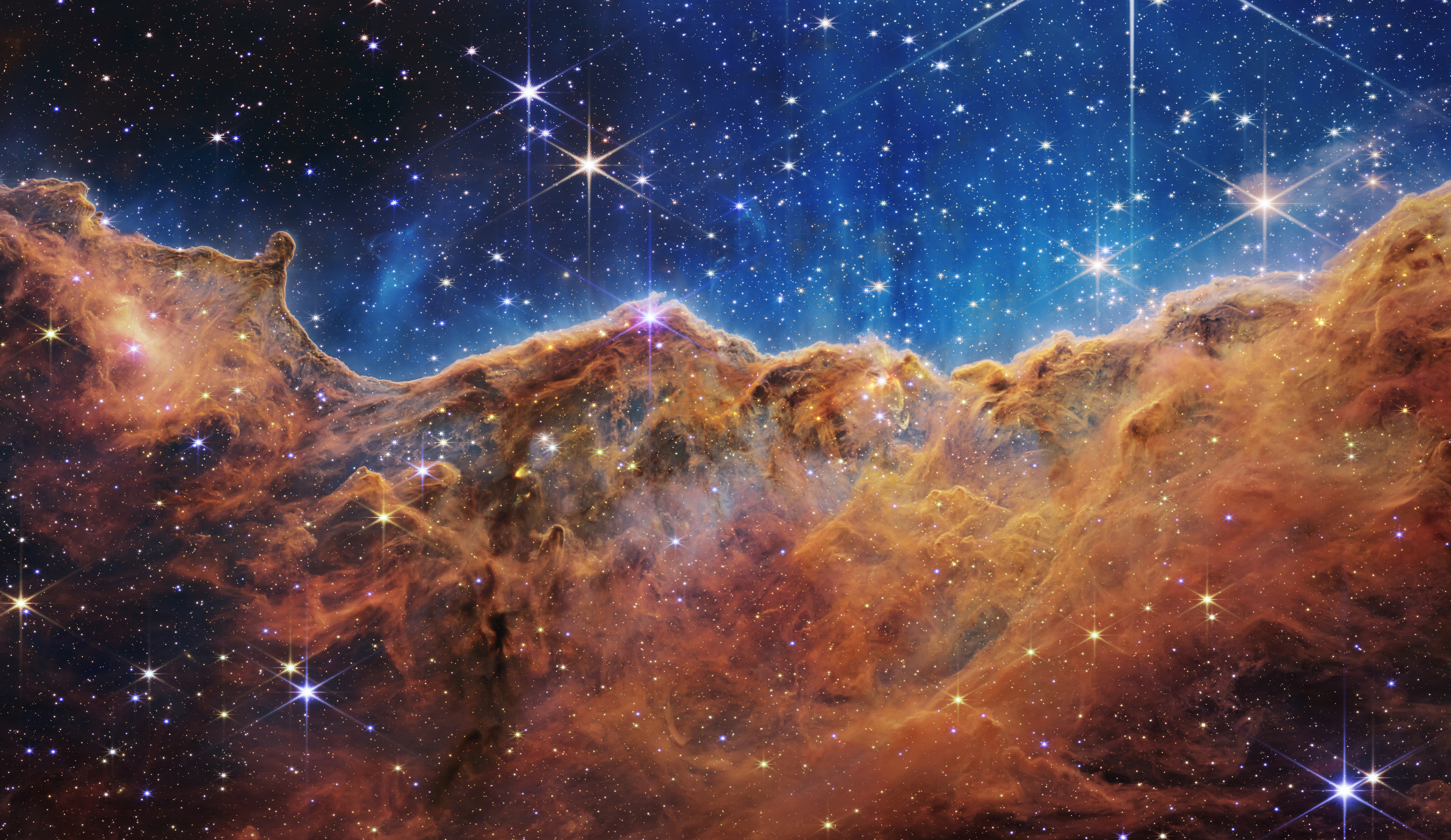
A data type is a classification of types of data that determines the possible values for that type, and the operations that can be performed on it.
There are several built-in data types in most programming languages, and you can also create your own custom data types using structures or classes.
Here are a few examples of common data types:
-
Integer: An integer is a whole number that can be positive, negative, or zero. For example: 10, -5, 0.
-
Floating-point: A floating-point number is a number with a decimal point. It is often used to represent fractional values or values that are too large or small to be represented as an integer. For example: 3.14, -2.718, 1.0e10.
-
String: A string is a sequence of characters, such as a word or a phrase. Strings are usually enclosed in quotes. For example: "hello", "goodbye", "Hello, world!".
-
Boolean: A boolean is a data type that has only two values: true and false. Booleans are often used in control structures to make decisions based on the value of a condition.
-
Array: An array is a data type that stores a collection of values. Arrays are indexed by integers and can be used to store values of any data type.
-
Object: An object is a data type that represents a real-world entity or concept. Objects are often used to model complex data structures and are created using classes.
Different data types have different properties and behaviors, and you can perform different operations on them. For example, you can add two integers together, but you cannot concatenate two integers the way you can concatenate two strings. Understanding data types is an important concept in programming and is essential for writing correct and efficient code.
- Type coercion: Some programming languages allow you to perform operations on values of different data types. In these cases, the values are often automatically converted, or "coerced," to the appropriate data type. For example, you might be able to add an integer and a floating-point number together, and the integer will be coerced to a floating-point number before the operation is performed.
x = 10
y = 3.14
# The integer "x" is coerced to a floating-point number before the operation is performed
z = x + y # z is now 13.14
# The string "hello" is coerced to a floating-point number (which results in an error)
a = "hello" + y # This will give an error
- Type conversion: Type conversion, also known as type casting, is the process of explicitly converting a value from one data type to another. Most programming languages provide functions or operators that allow you to perform type conversion. For example, in Python you can use the
int()
function to convert a floating-point number to an integer, or thestr()
function to convert an integer to a string.
x = 10
y = 3.14
# Convert "x" to a floating-point number
z = float(x) # z is now 10.0
# Convert "y" to an integer (the decimal part will be truncated)
a = int(y) # a is now 3
# Convert "x" to a string
b = str(x) # b is now "10"
- Type inference: Some programming languages, such as Python and Swift, have type inference systems that can automatically determine the data type of a value based on how it is used. This can make it easier to write code, because you don't always have to specify the data type of a value explicitly.
# The type of "x" is inferred to be an integer
x = 10
# The type of "y" is inferred to be a floating-point number
y = 3.14
# The type of "z" is inferred to be a string
z = "hello"
- Custom data types: In addition to the built-in data types provided by a programming language, you can also create your own custom data types using structures or classes. This can be useful for modeling complex data structures or for creating reusable components.
# Define a custom data type "Person" using a class
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def say_hello(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
# Create an instance of the "Person" data type
p = Person("Alice", 30)
# Call a method on the "p" object
p.say_hello() # Output: "Hello, my name is Alice and I am 30 years old."