Functions
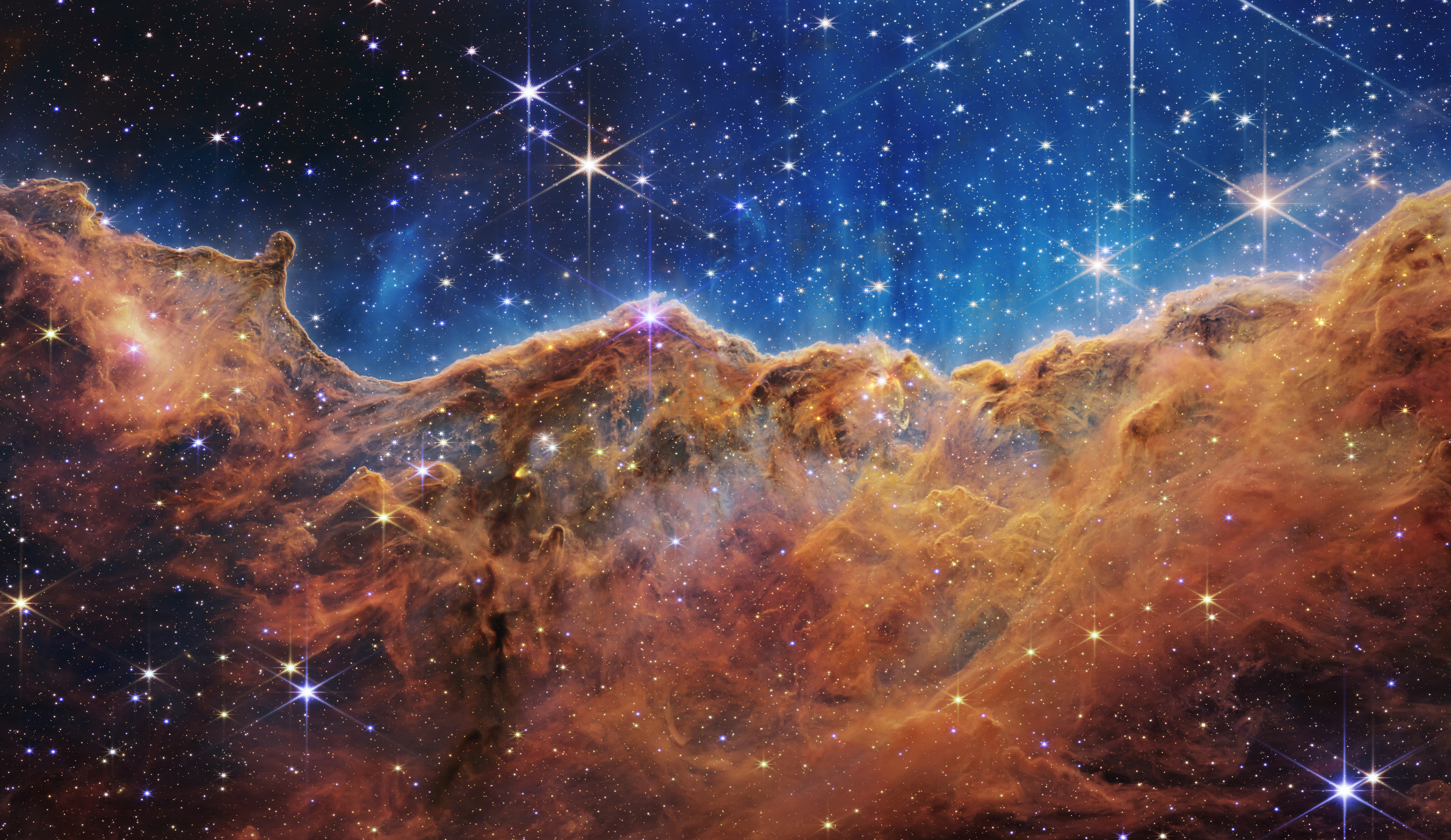
Functions are a fundamental concept in programming that allow for the organization and reuse of code. A function is a block of code that performs a specific task, and it can be called by other parts of the code as many times as needed. Functions are also known as procedures or subroutines in some languages.
Functions are useful for several reasons:
-
They provide a way to organize and structure code, making it more readable and maintainable.
-
They allow for the reuse of code, which helps to reduce redundancy and improve efficiency.
-
They allow for the separation of concerns, which means that different parts of the code can focus on different tasks without interfering with each other.
A function is a block of code that performs a specific task and may return a value. Functions are a way to organize and reuse code, and they allow you to write code that is more modular and easier to understand and maintain.
A function typically takes some input (called arguments) and produces an output (called the return value). The input and output of a function can vary. Some functions don't take any arguments and don't return a value. Others might take multiple arguments and return multiple values.
Here is an example of a simple function in Python:
def greet(name):
print("Hello, " + name + "!")
greet("Alice") # Output: "Hello, Alice!"
greet("Bob") # Output: "Hello, Bob!"
In this example, we define a function called greet
that takes a single parameter called name
. The function prints a greeting message to the console.
We can then call the function by passing it a value for the name
parameter (e.g. greet("Alice")
). The function will execute the code inside the function body, using the value of the name
parameter to determine what to print.
Functions can also return a value to the caller using the return
statement. For example:
def add(x, y):
return x + y
result = add(2, 3) # result is set to 5
In this example, we define a function called add that takes two parameters, x
and y
, and returns the sum of those two values. We can then call the function and assign the result to a variable (e.g. result = add(2, 3)
).
Here are a few benefits of using functions in programming:
-
Reuse of code: Functions allow you to reuse code, which makes it easier to write and maintain. You can define a function once, and then use it multiple times in your program. This can save a lot of time and effort, especially for tasks that are repeated often. Functions can be called from multiple places in your program, which makes it easier to reuse code. This can be especially helpful if you have a piece of code that performs a common task and you want to use it in multiple places in your program.
-
Modularity: Functions allow you to divide your code into logical blocks, which makes it easier to understand and debug. You can think of each function as a small piece of a puzzle that fits together with other functions to solve a larger problem. This can be especially helpful for large programs, where it can be difficult to see the big picture without breaking the code down into smaller pieces.
-
Abstraction: Functions can help you abstract away the details of a specific task, making it easier to focus on the big picture. For example, you might use a function to sort a list of numbers, without worrying about the specific details of how the sorting algorithm works.
-
Testing and debugging: Functions can make it easier to test and debug your code. You can write test cases for individual functions and make sure they are working correctly, before integrating them into the larger program.
-
Improved readability: Functions can make your code more readable by giving descriptive names to blocks of code. This can make it easier for other people (or even yourself) to understand what your code does, especially for larger programs.
-
Easier code maintenance: Functions allow you to make changes to your code in a modular way. If you need to change something about the way a function works, you can make the change in one place (the function definition) instead of having to hunt down every instance of the code in your program.
Overall, using functions can make your code more readable, organized, debug, and maintainable.