Datetime
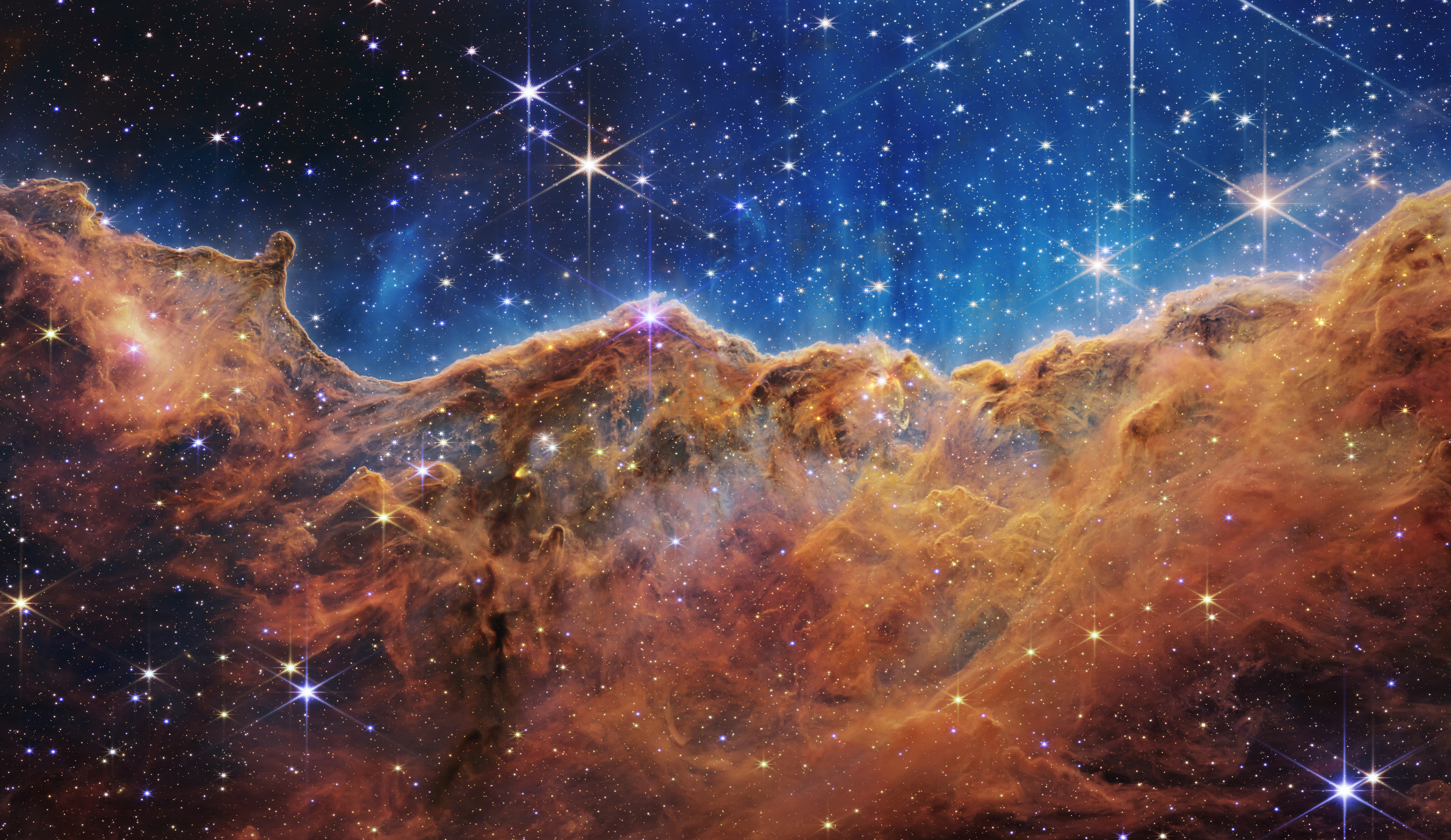
In programming, datetime
refers to a module or library that provides classes for working with dates and times. The datetime
module is a built-in module in Python, and it defines several classes for representing and manipulating dates and times.
A datetime
object represents a single point in time, and it stores the date and time as a tuple of integers (year, month, day, hour, minute, second, microsecond). You can use the datetime
module to create datetime
objects, extract the date and time components, and perform arithmetic with datetime
objects.
In Python, the datetime
module provides classes for working with dates and times. The datetime
module defines several classes, including:
-
datetime
: represents a single point in time. It stores the date and time as a tuple of integers (year, month, day, hour, minute, second, microsecond). -
date
: represents a specific calendar date (year, month, day). -
time
: represents a specific time of day (hour, minute, second, microsecond). -
timedelta
: represents a duration, which can be used to perform arithmetic with datetime objects.
Here is an example of how to use the datetime
module in Python:
from datetime import datetime
# Create a datetime object for the current date and time
now = datetime.now()
print(now) # Output: "2022-01-02 13:14:15.161718" (example)
# Extract the date and time components
year = now.year
month = now.month
day = now.day
hour = now.hour
minute = now.minute
second = now.second
microsecond = now.microsecond
# Create a datetime object for a specific date and time
new_year = datetime(2022, 1, 1, 0, 0, 0)
# Calculate the difference between two datetime objects
difference = now - new_year
print(difference) # Output: "1 day, 13:14:15.161718" (example)
To format a date in most programming languages, you can use a library or module that provides functions for formatting dates and times.
For example, in Python, you can use the datetime
module to create a datetime
object representing a specific date and time, and then use the strftime function to format the date and time according to a specific format string.
Here is an example of how to format a date in Python:
from datetime import datetime
# Create a datetime object for the current date and time
now = datetime.now()
# Use the strftime function to format the date and time
date_string = now.strftime("%Y-%m-%d") # format as YYYY-MM-DD
time_string = now.strftime("%H:%M:%S") # format as HH:MM:SS
print(date_string) # Output: "2022-01-02" (example)
print(time_string) # Output: "13:14:15" (example)
In this example, we create a datetime
object representing the current date and time using the datetime.now()
function. We then use the strftime function to format the date and time as strings. The %Y, %m, %d
,
To convert a string to a datetime
object in Python, you can use the datetime.strptime
function, which takes a string and a format string as arguments, and returns a datetime
object.
Here is an example of how to use the datetime.strptime
function in Python:
from datetime import datetime
# Convert a string to a datetime object
date_string = "2022-01-02"
date_format = "%Y-%m-%d"
date = datetime.strptime(date_string, date_format)
print(date) # Output: "2022-01-02 00:00:00"
# Convert a string to a datetime object with a time component
datetime_string = "2022-01-02 13:14:15"
datetime_format = "%Y-%m-%d %H:%M:%S"
datetime = datetime.strptime(datetime_string, datetime_format)
print(datetime) # Output: "2022-01-02 13:14:15"
In these examples, we use the datetime.strptime
function to parse a string and convert it to a datetime
object. The date_format
and datetime_format
strings specify the format of the input string. The function uses these format strings to parse the string and extract the date and time components.