Constraints
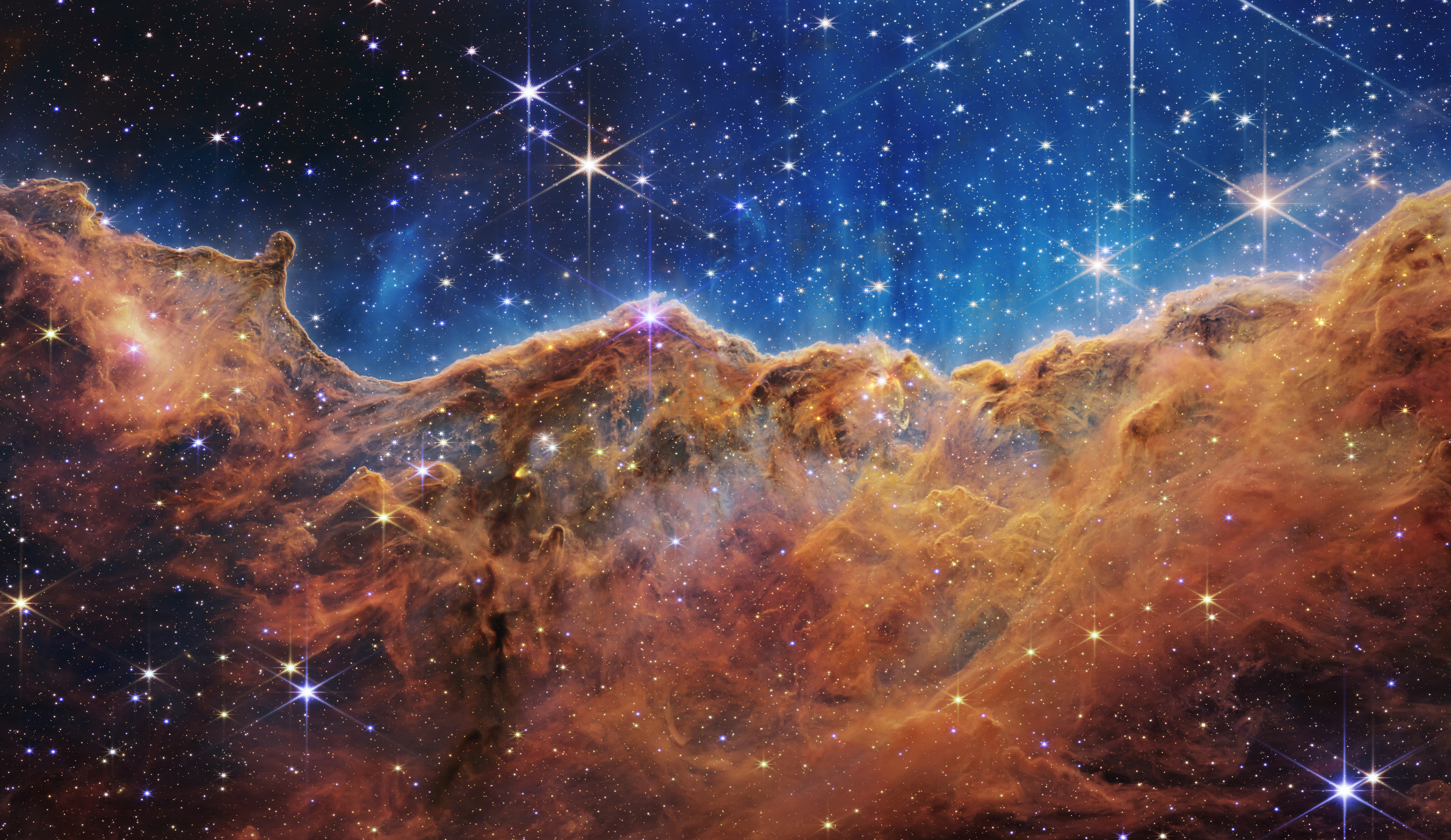
Constraints are limitations or restrictions that are placed on a system or process. In programming, constraints can be used to enforce rules or limits on the input, output, or behavior of a program.
Here are a few examples of constraints that might be used in programming:
-
Input constraints: These are limits on the data that can be input into a program. For example, you might have a program that only accepts integers as input and rejects any other data type.
-
Output constraints: These are limits on the data that can be output by a program. For example, you might have a program that only generates numbers within a certain range, or a program that only generates strings with a certain length.
-
Resource constraints: These are limits on the resources that a program can use. For example, you might have a program that is only allowed to use a certain amount of memory, or a program that is only allowed to run for a certain amount of time.
-
Design constraints: These are limitations that are imposed on the design of a program. For example, you might have a program that is required to be written in a certain programming language, or a program that is required to follow a certain design pattern.
-
Constraints can be used to ensure that a program behaves in a predictable and controlled way. They can also help to prevent errors and make it easier to debug and maintain your code.
Here are a few examples of constraints in Python:
# A function that only accepts integers as input and returns the input value squared
def square(x: int) -> int:
return x * x
# A function that only accepts strings as input and returns a string with all uppercase letters
def to_upper(s: str) -> str:
return s.upper()
# A function that only returns values between 0 and 100
def clamp(x: int) -> int:
if x < 0:
return 0
elif x > 100:
return 100
else:
return x
In this example, the square
function takes an integer as input (x
) and returns the input value squared. The to_upper
function takes a string as input (s
) and returns a new string with all uppercase letters. The clamp
function takes an integer as input (x
) and returns a value between 0 and 100, depending on the value of x
.
The types of the input and output for each function are specified using type hints. For example, square
is defined as a function that takes an integer (int
) as input and returns an integer (int
) as output.
The input and output constraints for each function are enforced by the type hints. If you try to call the square
function with a non-integer value, or the to_upper
function with a non-string value, you will get an error. Similarly, the clamp
function is only allowed to return values between 0 and 100.
Overall, these constraints help to ensure that the functions behave in a predictable and controlled way, and they make it easier to understand and use the functions.