Hashmap
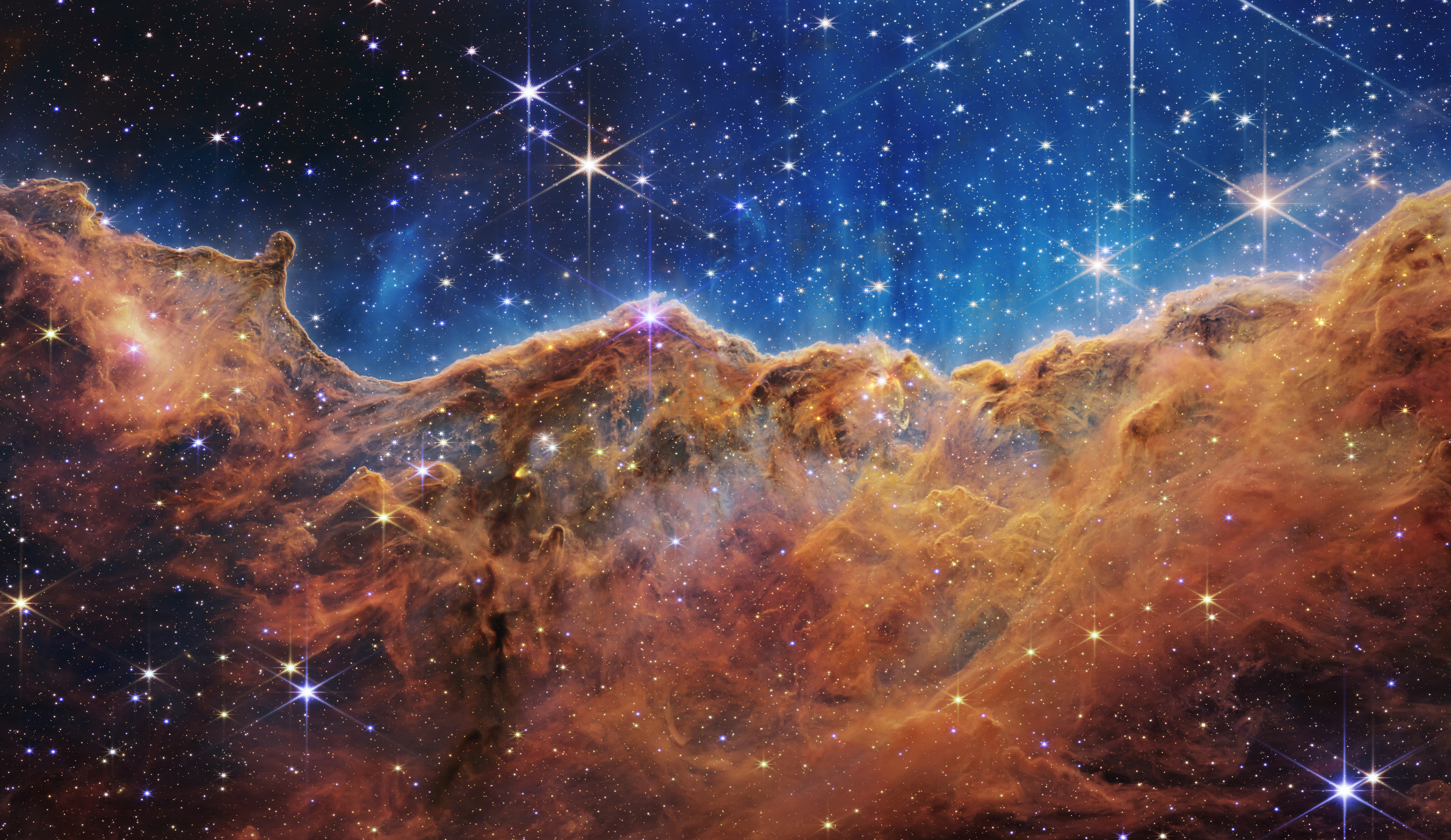
A hashmap, also known as a hash table, is a data structure that maps keys to values. It is an efficient way to store and retrieve data, and is often used in computer programs to implement associative arrays, symbol tables, and other data structures.
A hashmap works by using a hash function to map keys to indexes in an array. When you want to add a key-value pair to the hashmap, the hash function is used to calculate the index at which the value should be stored. To retrieve a value, the hash function is used again to calculate the index, and the value is retrieved from that location.
One advantage of a hashmap is that it allows for fast lookups and insertions, since it can calculate the index of a value in constant time. However, a disadvantage is that the order of the elements in the hashmap is not preserved, and the performance of a hashmap can degrade if the hash function does not distribute the keys evenly.
Here is an example of a simple hashmap in Python that maps strings (the keys) to integers (the values):
# create a new hashmap
map = {}
# add some key-value pairs to the hashmap
map["apple"] = 1
map["banana"] = 2
map["cherry"] = 3
# retrieve a value by its key
banana_value = map["banana"] # banana_value will be 2
In this example, we create a new empty dictionary called map
and add three key-value pairs to it: "apple" maps to 1, "banana" maps to 2, and "cherry" maps to 3. Then, we retrieve the value associated with the key "banana", which is 2.
Note that a dictionary in Python is an implementation of a hashmap, and it works in a similar way as the example in Java. Dictionaries are not ordered data structures, so the elements are not stored in the order they were added. The order of the elements in a dictionary may also change when elements are added or removed.