Lambda
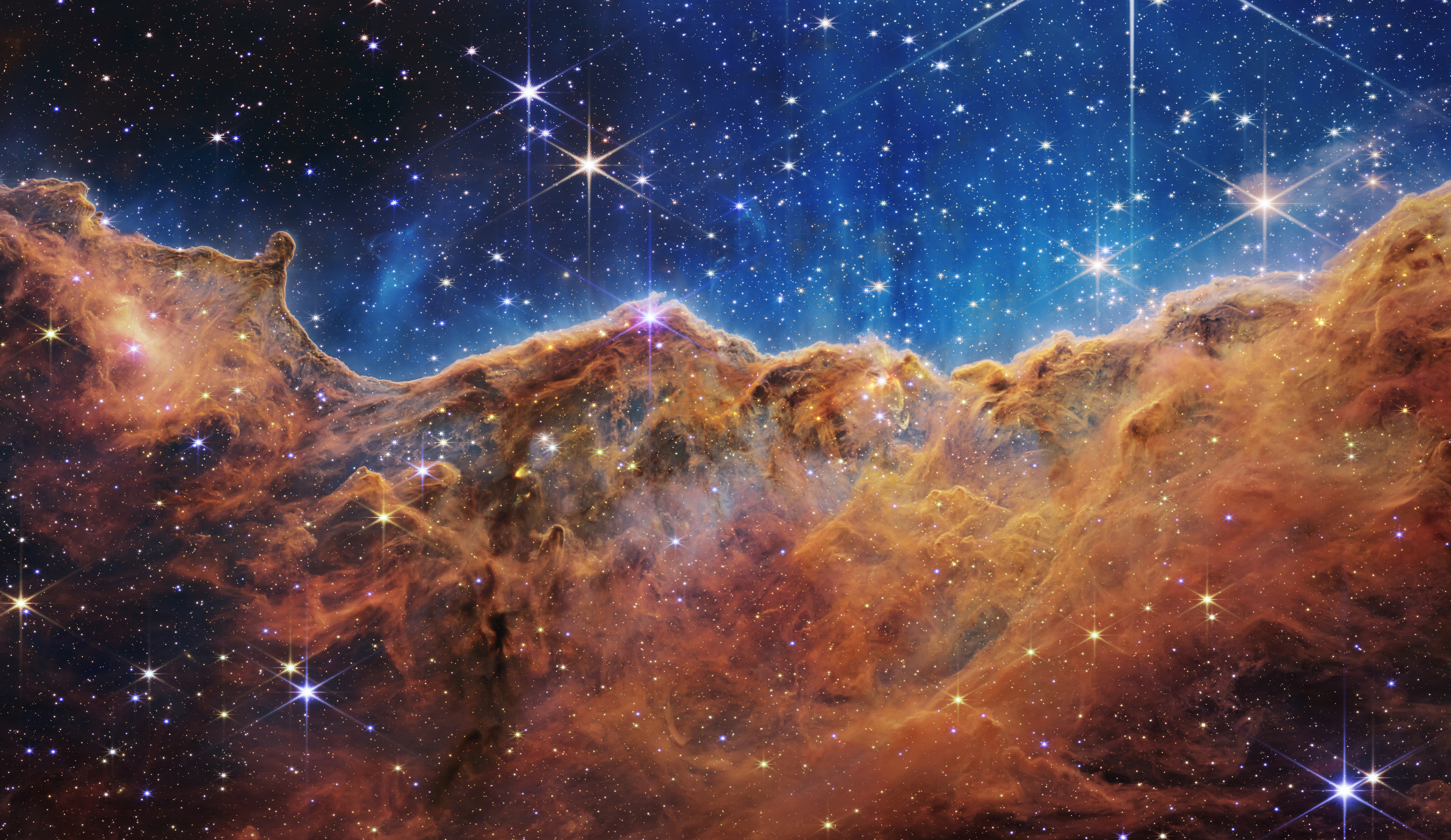
In Python and some other programming languages, a lambda function is a small anonymous function without a name. It is defined using the lambda
keyword and has the following syntax:
lambda arguments: expression
Lambda functions are often used as a simple way to create small functions on the fly, and they are particularly useful in the context of higher-order functions (functions that take other functions as arguments).
Here is an example of how to use a lambda function in Python:
# Define a lambda function that adds two numbers
add = lambda x, y: x + y
# Call the lambda function
result = add(3, 4) # result is 7
# Use the lambda function as an argument to another function
numbers = [1, 2, 3, 4, 5]
sorted_numbers = sorted(numbers, key=lambda x: -x) # sort the numbers in descending order
print(sorted_numbers) # Output: [5, 4, 3, 2, 1]
In this example, we define a lambda function called add
that takes two arguments, x
and y
, and returns their sum. We then call the lambda function and pass it two arguments, and it returns the sum.
We also use the lambda function as an argument to the built-in sorted
function, which sorts a list of numbers in ascending order by default. By using the lambda x: -x
function as the key
argument, we sort the numbers in descending order instead.