Loops
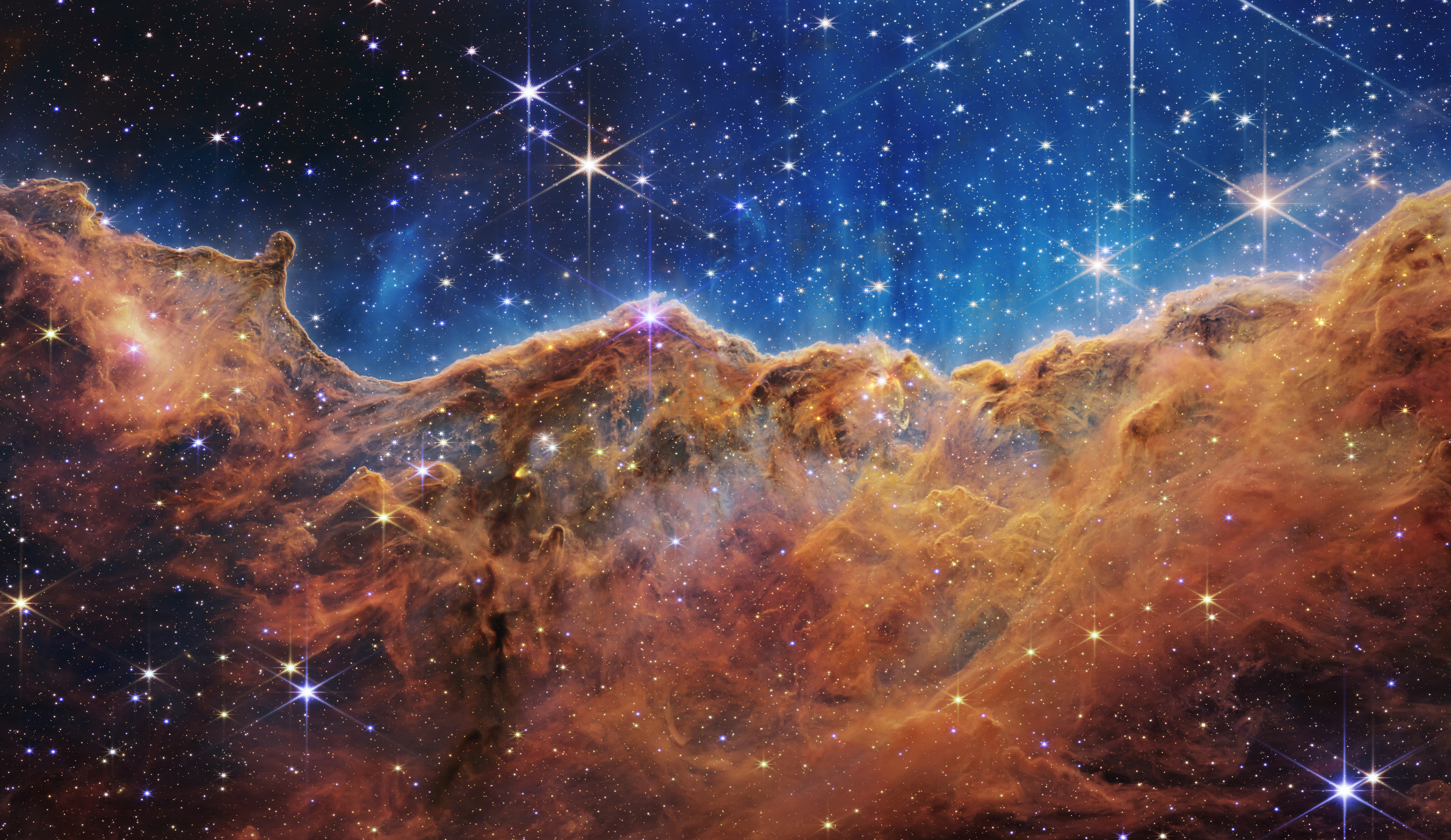
In programming, loops are used to execute a block of code repeatedly until a certain condition is met. There are several types of loops that you can use in different programming languages:
-
for
loops: Afor
loop is used to iterate over a sequence (such as a list, tuple, or string) or other iterable object. The loop variable takes on each value in the sequence, and the code inside the loop is executed for each value. -
while
loops: Awhile
loop is used to repeat a block of code as long as a certain condition is true. The condition is checked at the beginning of each iteration, and the loop will continue to execute as long as the condition is true. -
do-while
loops: Ado-while
loop is similar to awhile
loop, except that the condition is checked at the end of each iteration instead of at the beginning. This means that the code inside the loop will be executed at least once, even if the condition is initially false. -
foreach
loops: Aforeach
loop is a type of loop that is available in some programming languages (such as PHP and C#). It is used to iterate over the elements of an array or other iterable object, and the loop variable takes on each value in the sequence.
A for loop is a control flow statement that allows you to iterate over a sequence (such as a list, tuple, or string) or other iterable object and execute a block of code for each item in the sequence.
Here is an example of a simple for loop in Python:
for i in range(5):
print(i)
# Output:
# 0
# 1
# 2
# 3
# 4
In this example, the for loop iterates over the values in the range(5) object, which generates a sequence of integers from 0 to 4. The loop variable i takes on each value in the sequence, and the code inside the loop is executed for each value.
Here is another example that loops over a list of strings:
colors = ["red", "green", "blue"]
for color in colors:
print(color)
# Output:
# red
# green
# blue
In this example, the for loop iterates over the values in the colors list, and the loop variable color takes on each value in the list. The code inside the loop is executed for each value.