Tensorflow
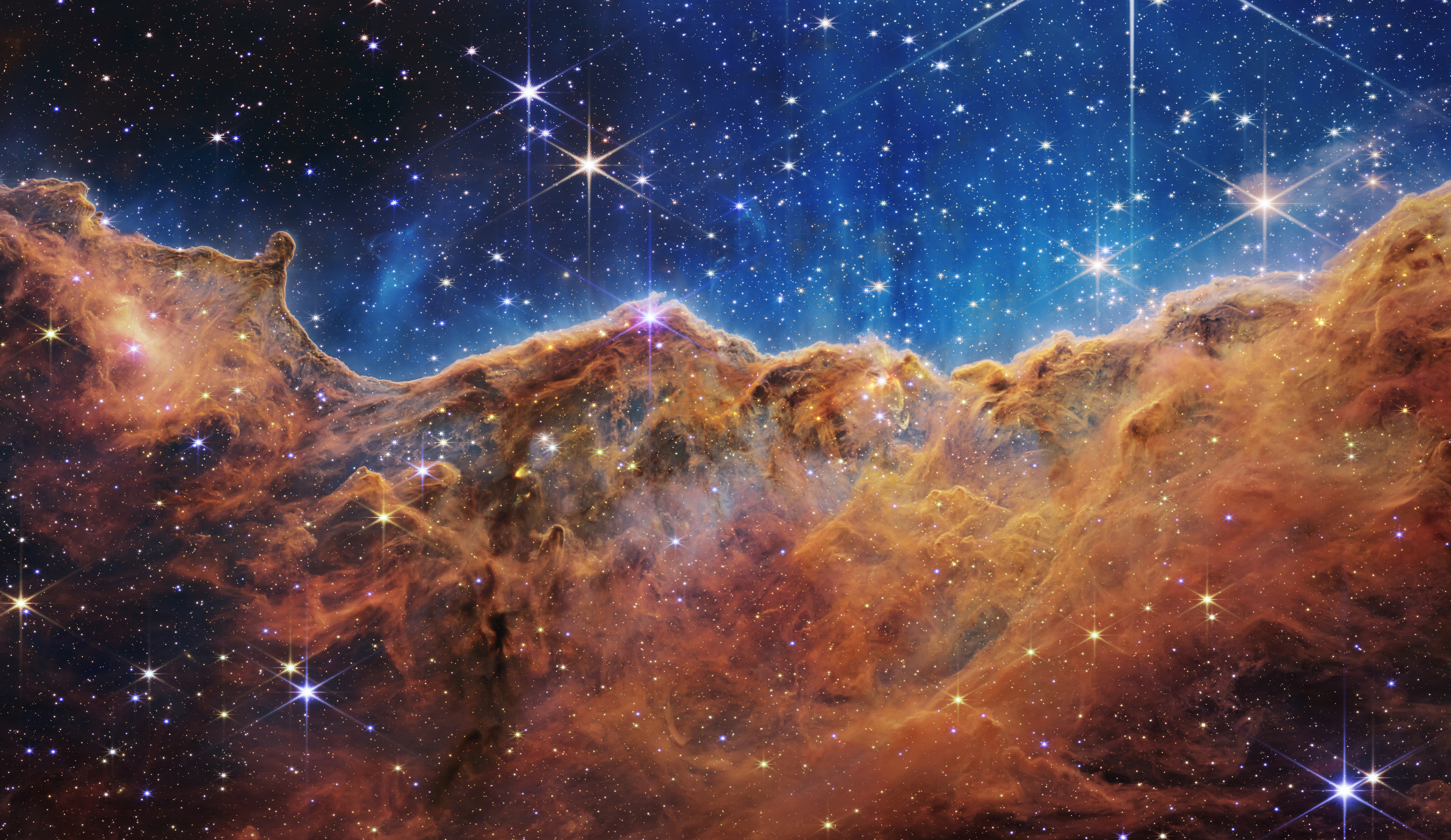
TensorFlow is an open-source software library for machine learning and artificial intelligence. It was developed by Google and is widely used in industry and academia for tasks such as training and deploying machine learning models, building neural networks, and analyzing and visualizing data.
TensorFlow is based on the concept of a computational graph, which is a series of operations that are performed on tensors (multi-dimensional arrays). TensorFlow allows you to build and execute these computational graphs, and provides a variety of functions and tools for machine learning and data analysis.
TensorFlow is available in Python and C++, and it can be used on a variety of platforms, including desktop, mobile, and cloud. It is a powerful and flexible tool for building and training machine learning models.
Here is a simple example of how to use TensorFlow to fit a linear regression model to a set of data:
import tensorflow as tf
# Generate some synthetic data
x_data = [1, 2, 3, 4, 5]
y_data = [1, 2, 3, 4, 5]
# Define the model
w = tf.Variable(0.0, name='weights')
b = tf.Variable(0.0, name='bias')
def model(x):
return w * x + b
# Define the loss function
def loss(y_pred, y):
return tf.reduce_mean(tf.square(y_pred - y))
# Define the optimizer
optimizer = tf.optimizers.SGD(learning_rate=0.01)
# Define a metric to track the accuracy of the model
accuracy = tf.metrics.MeanSquaredError()
# Training loop
for epoch in range(100):
with tf.GradientTape() as tape:
y_pred = model(x_data)
current_loss = loss(y_pred, y_data)
gradients = tape.gradient(current_loss, [w, b])
optimizer.apply_gradients(zip(gradients, [w, b]))
accuracy(y_pred, y_data)
print(f'Epoch {epoch}, Loss: {current_loss}, Accuracy: {accuracy.result()}')
# Evaluate the model on new data
x_new = [6, 7, 8]
y_new = model(x_new)
print(y_new)
In this example, we use TensorFlow to define a linear regression model, a loss function, and an optimizer. We then use a gradient descent optimizer to fit the model to the data. We also track the accuracy of the model using a mean squared error metric. Finally, we use the trained model to make predictions on new data.