K-means
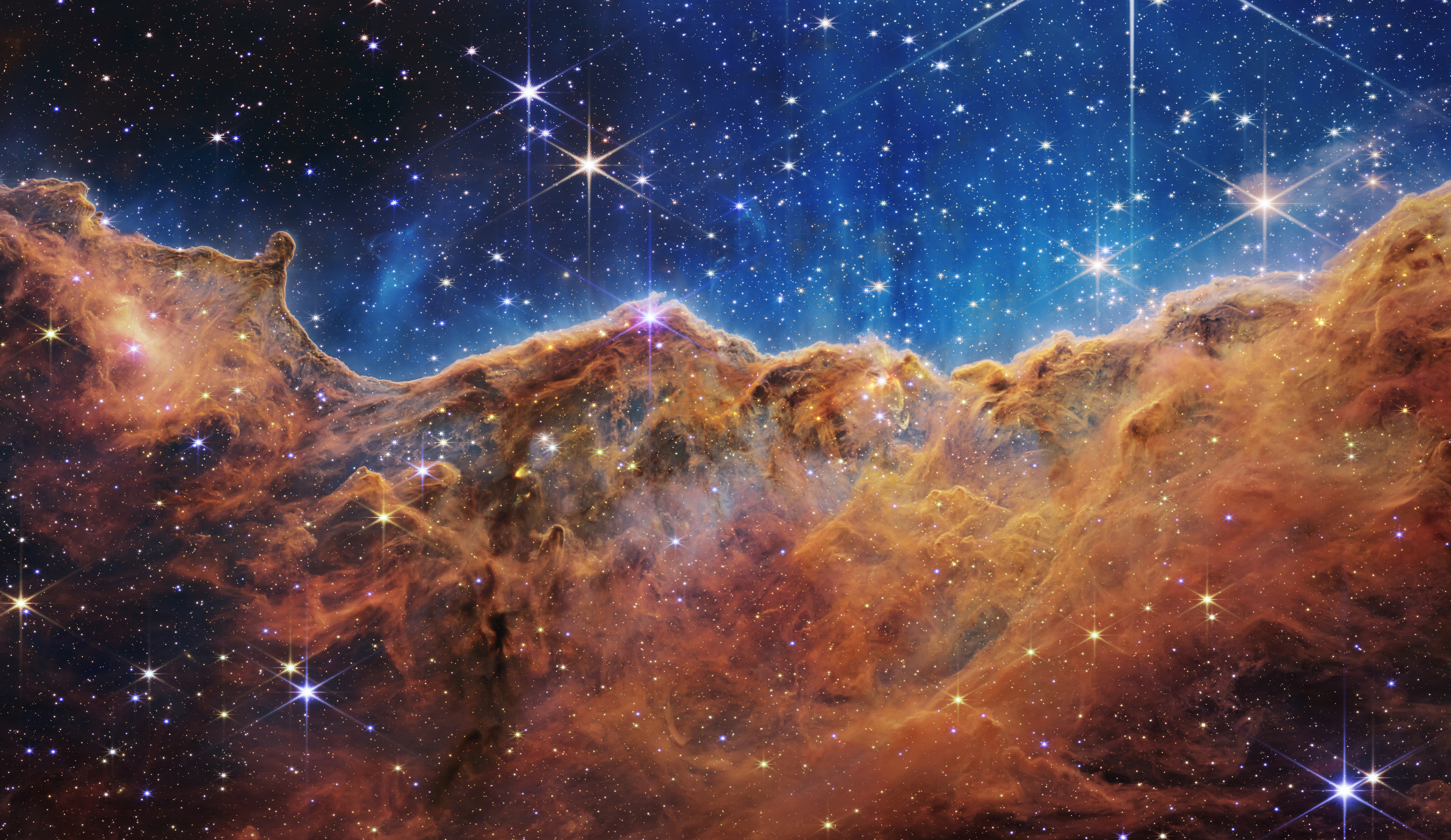
K-means is a popular centroid-based clustering algorithm used to group similar data points together into clusters.
The basic idea of the k-means algorithm is to partition a dataset of n points into k clusters, where k is a user-specified parameter. The algorithm starts by randomly selecting k centroids, and then it iteratively assigns each data point to the cluster with the nearest centroid. The centroids are then recomputed as the mean of the points in each cluster. The algorithm stops when the assignment of points to clusters no longer changes.
The k-means algorithm can be summarized in the following steps:
Initialize k centroids randomly. Assign each data point to the cluster that has the nearest centroid. Recompute the centroids as the mean of the points in each cluster. Repeat steps 2 and 3 until the assignment of points to clusters no longer changes. The k-means algorithm is sensitive to the initial placement of the centroids, so it's common to run the algorithm multiple times with different initial centroids. The final result is the best one among all the trial.
K-means is a simple and fast algorithm, it's easy to understand and implement, and it's widely used in many fields such as data mining, image processing, and bioinformatics. However, k-means has some limitations. For instance, k-means assumes that clusters are spherical and equally sized, which may not be the case in some datasets. Also, k-means can get stuck in local optima, which means that the final solution may not be the global optimum.
An example of using TensorFlow to implement a clustering algorithm is using the k-means algorithm to cluster a dataset of points in 2D space. Here is an example of how to implement k-means using TensorFlow:
import tensorflow as tf
import numpy as np
# Generate a dataset of points
num_points = 1000
points = np.random.uniform(low=-10, high=10, size=(num_points, 2))
# Define the number of clusters
k = 4
# Initialize the centroids randomly
centroids = tf.Variable(tf.slice(tf.random.shuffle(points), [0, 0], [k, -1]))
# Create the placeholders for the points and the centroids
points_ph = tf.placeholder(tf.float32, [None, 2])
centroids_ph = tf.placeholder(tf.float32, [k, 2])
# Compute the distances between the points and the centroids
distances = tf.reduce_sum(tf.square(points_ph[:, tf.newaxis] - centroids_ph), axis=2)
# Find the nearest centroid for each point
nearest_indices = tf.argmin(distances, axis=1)
# Group the points by centroid
gathered_points = tf.gather(points_ph, nearest_indices)
# Compute the new centroids as the mean of the points in each cluster
means = tf.reduce_mean(gathered_points, axis=0)
# Create an operation to update the centroids
update_centroids = tf.assign(centroids, means)
# Initialize the variables
init = tf.global_variables_initializer()
# Run the k-means algorithm
with tf.Session() as sess:
sess.run(init)
for i in range(100):
sess.run(update_centroids, feed_dict={points_ph: points, centroids_ph: centroids.eval()})
clusters = sess.run(nearest_indices, feed_dict={points_ph: points, centroids_ph: centroids.eval()})
final_centroids = centroids.eval()