Numpy
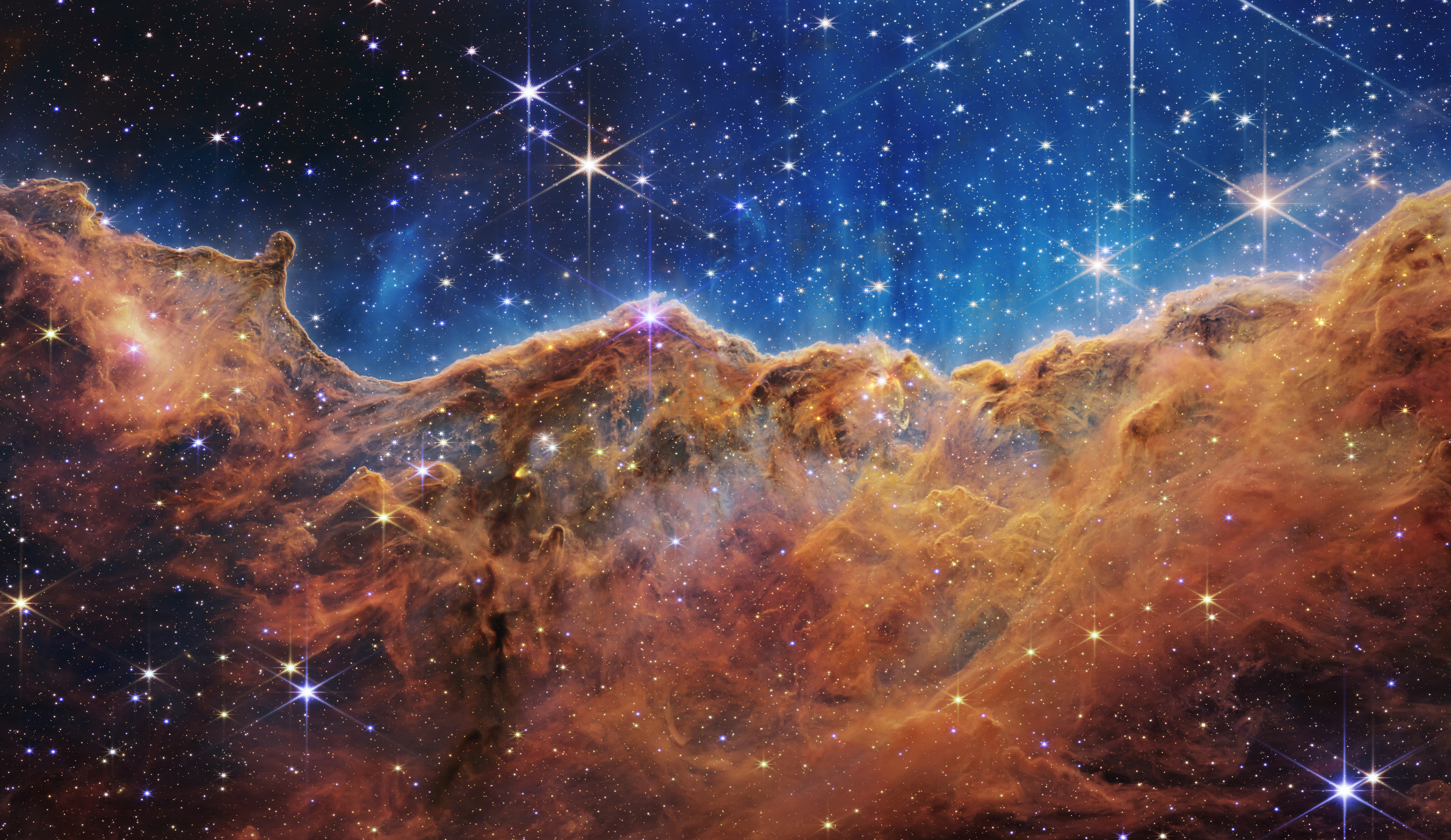
NumPy is a software library in Python that is used for scientific computing and data analysis. It provides support for large, multi-dimensional arrays and matrices of numerical data, and a large collection of mathematical functions to operate on these arrays.
One of the main data structures in NumPy is the ndarray
(n-dimensional array), which is a multi-dimensional array of homogeneous data (i.e., data of the same type, such as integers or floating point values). NumPy provides a variety of functions and methods for working with ndarray
s, including mathematical operations, sorting, and reshaping.
NumPy is widely used in the scientific and data science communities for tasks such as numerical simulation, statistical analysis, and machine learning. It is an essential package for working with numerical data in Python.
Here is an example of how to use NumPy to create an ndarray and perform some basic operations on it:
import numpy as np
# Create an array of zeros
a = np.zeros(3)
print(a) # Output: [0. 0. 0.]
# Create a 2x2 array of ones
b = np.ones((2, 2))
print(b) # Output: [[1. 1.]
# [1. 1.]]
# Create a 3x3 identity matrix
c = np.eye(3)
print(c) # Output: [[1. 0. 0.]
# [0. 1. 0.]
# [0. 0. 1.]]
# Create an array of random values
d = np.random.rand(3, 3)
print(d) # Output: [[0.73 0.59 0.38]
# [0.89 0.72 0.02]
# [0.73 0.02 0.53]]
# Perform element-wise multiplication of two arrays
e = b * d
print(e) # Output: [[0.73 0.59 0.38]
# [0.89 0.72 0.02]
# [0.73 0.02 0.53]]
# Perform matrix multiplication
f = np.dot(b, d)
print(f) # Output: [[1.49 1.29]
# [1.49 1.29]]
In this example, we use NumPy to create and manipulate ndarray
s. We use the zeros
, ones
, and eye
functions to create arrays with specific values, and the random.rand