Semi-supervised learning
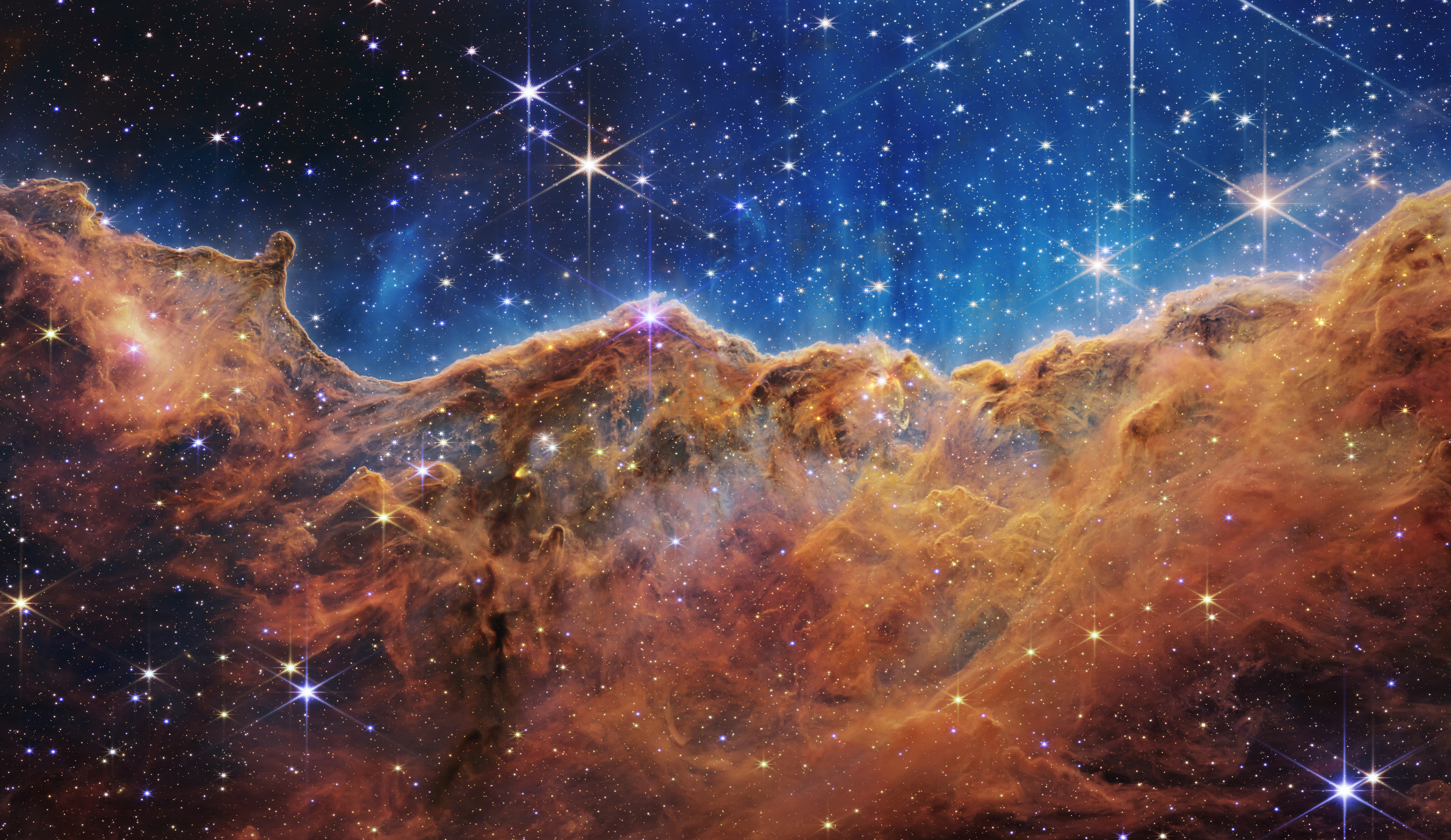
Semi-supervised learning is a type of machine learning that lies between supervised learning and unsupervised learning. In semi-supervised learning, the model is trained on a dataset that is partially labeled, with some examples in the training set having their correct output labels, and others being unlabeled.
Semi-supervised learning is useful in situations where it is expensive or time-consuming to label a large dataset, but a small amount of labeled data is still available. The model can use the labeled data to learn about the structure of the data, and can then use that knowledge to make predictions about the unlabeled data.
Some common applications of semi-supervised learning include natural language processing and image classification. In these cases, the model might be trained on a dataset that consists of a few thousand labeled examples and a much larger number of unlabeled examples. The model can then use the labeled examples to learn about the structure of the data, and can make predictions about the unlabeled examples using that knowledge.
There are many different algorithms that can be used for semi-supervised learning, including both supervised learning algorithms and unsupervised learning algorithms. The best choice for a particular problem will depend on the characteristics of the data and the requirements of the task.
Here are a few example use cases for semi-supervised learning:
-
Image classification: In a large dataset of images, it might be expensive or time-consuming to manually label all of the images. However, if a small number of labeled examples are available, a model trained using semi-supervised learning can use those labeled examples to learn about the structure of the data, and can then make predictions about the unlabeled examples using that knowledge.
-
Natural language processing: In a large dataset of text, it might be expensive or time-consuming to manually label all of the text. However, if a small number of labeled examples are available, a model trained using semi-supervised learning can use those labeled examples to learn about the structure of the data, and can then make predictions about the unlabeled examples using that knowledge.
-
Fraud detection: In a large dataset of financial transactions, it might be expensive or time-consuming to manually label all of the transactions as fraudulent or not fraudulent. However, if a small number of labeled examples are available, a model trained using semi-supervised learning can use those labeled examples to learn about the structure of the data, and can then make predictions about the unlabeled examples using that knowledge.
There are many different algorithms that can be used for semi-supervised learning, including both supervised learning algorithms and unsupervised learning algorithms. Some common algorithms include:
-
Support vector machines (SVMs): A supervised learning algorithm that can be used for classification tasks. SVMs can be trained on a dataset that is partially labeled, and can use the labeled data to learn about the structure of the data.
-
K-means clustering: An unsupervised learning algorithm that can be used for clustering tasks. K-means clustering can be used on a partially labeled dataset, and can use the labeled data to inform the clustering process.
-
Neural networks: A flexible model that can be used for both classification and regression tasks. Neural networks can be trained on a partially labeled dataset, and can use the labeled data to learn about the structure of the data.
-
Self-training: A simple semi-supervised learning algorithm that involves using a small number of labeled examples to train a model, and then using the model to label additional examples. The process is repeated until the model has been trained on a sufficient number of labeled examples.
This is just a small sampling of the many different algorithms that are available for semi-supervised learning. The best choice for a particular problem will depend on the characteristics of the data and the requirements of the task.
Here is an example of a simple semi-supervised learning model using TensorFlow:
import tensorflow as tf
# Load the data
(x_train, y_train), (x_test, y_test) = tf.keras.datasets.mnist.load_data()
# Normalize the data
x_train = x_train / 255.0
x_test = x_test / 255.0
# Flatten the data
x_train = x_train.reshape(-1, 28*28)
x_test = x_test.reshape(-1, 28*28)
# Split the labeled data into a training set and a validation set
x_labeled, y_labeled = x_train[:1000], y_train[:1000]
x_unlabeled, y_unlabeled = x_train[1000:], y_train[1000:]
# Build the model
input_layer = tf.keras.layers.Input(shape=(28*28,))
encoded = tf.keras.layers.Dense(128, activation='relu')(input_layer)
encoded = tf.keras.layers.Dense(64, activation='relu')(encoded)
decoded = tf.keras.layers.Dense(128, activation='relu')(encoded)
decoded = tf.keras.layers.Dense(28*28, activation='sigmoid')(decoded)
output_layer = tf.keras.layers.Dense(10, activation='softmax')(encoded)
autoencoder = tf.keras.models.Model(input_layer, decoded)
classifier = tf.keras.models.Model(input_layer, output_layer)
# Compile the models
autoencoder.compile(optimizer='adam', loss='binary_crossentropy')
classifier.compile(optimizer='adam', loss='sparse_categorical_crossentropy', metrics=['accuracy'])
# Train the autoencoder on the unlabeled data
autoencoder.fit(x_unlabeled, x_unlabeled, epochs=5)
# Train the classifier on the labeled data
classifier.fit(x_labeled, y_labeled, epochs=5)
# Evaluate the classifier on the test set
test_loss, test_acc = classifier.evaluate(x_test, y_test)
In this example, we are using a neural network autoencoder to learn a low-dimensional representation of the MNIST dataset, and then using that representation to train a supervised classifier. The autoencoder is trained on the unlabeled data and the classifier is trained on the labeled data.
The autoencoder is a neural network with two parts: an encoder, which maps the input data to a lower-dimensional representation, and a decoder, which maps the lower-dimensional representation back to the original data space. During training, the autoencoder is presented with the unlabeled data and attempts to reconstruct those examples using the encoder and decoder. The model is trained using binary crossentropy loss, which measures the difference between the reconstructed examples and the original examples.
The classifier is a simple feedforward neural network with two layers: a fully-connected (dense) layer with 64 units and an output layer with 10 units, one for each digit class. The input to the classifier is the low-dimensional representation of the data learned by the autoencoder, and the output is a probability distribution over the 10 digit classes. The classifier is trained using sparse categorical crossentropy loss, which measures the difference between the predicted probability distribution and the true probability distribution.
After training, the classifier can be used to make predictions on new, unseen examples. To evaluate the classifier's performance, we can use the classifier.evaluate method to calculate the loss and accuracy on a separate test set. The test accuracy gives us an idea of how well the classifier is able to generalize to new examples.