Best practices
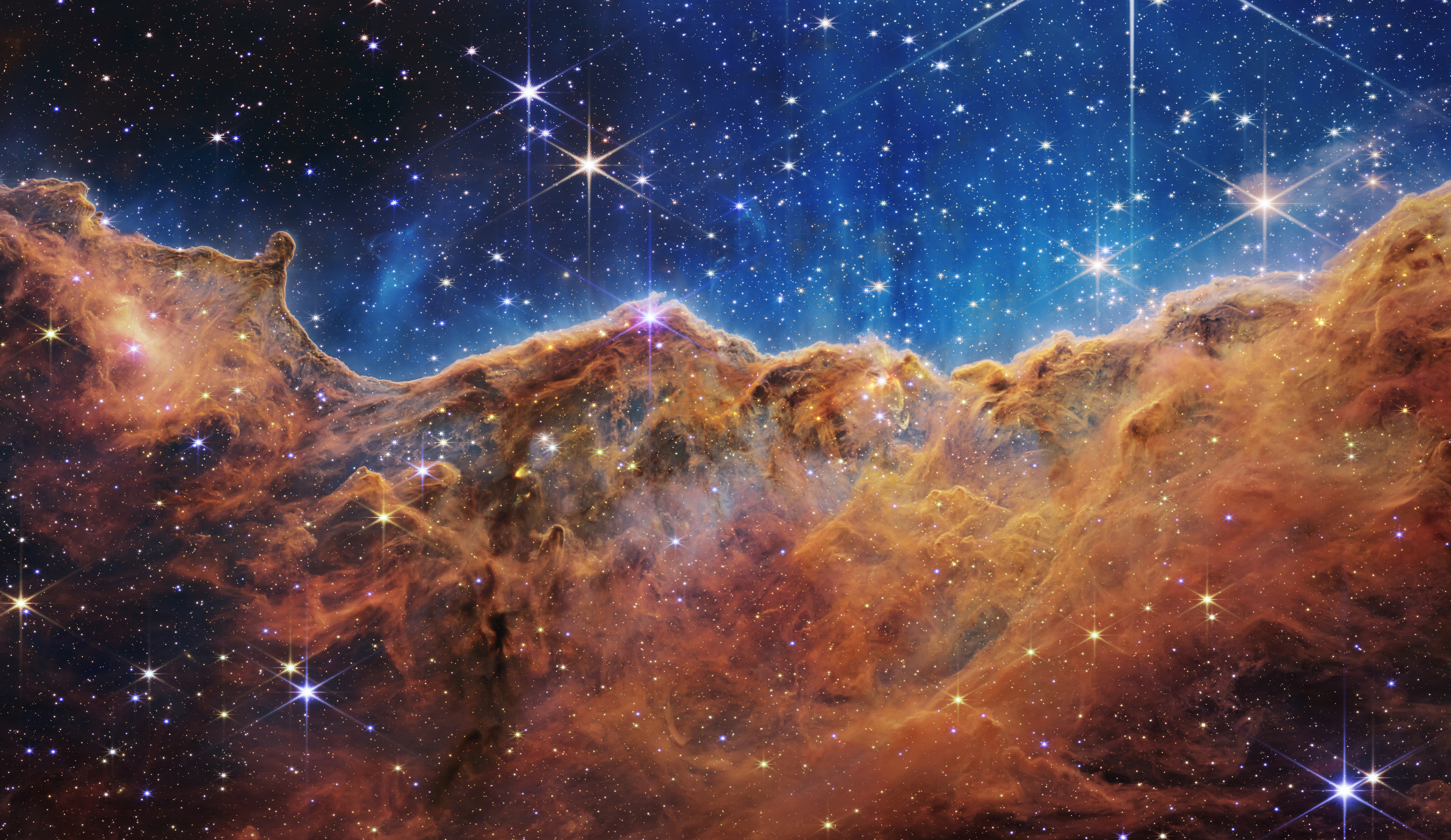
Best practices are guidelines or recommendations for designing and implementing software programs in a way that will result in high-quality, reliable, and maintainable code. Some common best practices in software development include:
Write clean, readable, and well-documented code
Clean code is easy to read and understand, and is free from unnecessary complexity and clutter. It follows consistent formatting conventions and uses descriptive and meaningful names for variables, functions, and other code elements. Well-documented code includes clear and concise comments explaining the purpose and usage of the code.
# Good example:
def calculate_mean(values):
"""
Calculate the mean of a list of values.
Parameters:
values (list): A list of numerical values.
Returns:
float: The mean of the values.
"""
total = sum(values)
count = len(values)
return total / count
# Bad example:
def calc_mean(v):
t = sum(v)
c = len(v)
return t / c
Use version control
Version control systems such as Git allow developers to track changes to the code, revert to previous versions, and collaborate with other developers. By keeping a record of every change to the code, version control systems make it easy to track the history of the code, identify and fix errors, and collaborate with other developers.
# Initialize a Git repository and add the code files
git init
git add .
# Make a commit with a descriptive message
git commit -m "Initial commit"
# Push the code to a remote repository
git push origin master
Use testing and debugging techniques
Testing and debugging techniques can help developers find and fix errors in the code. Unit testing involves writing small, independent test cases to validate the behavior of specific code units, such as functions or classes. Debugging tools, such as debuggers and print statements, allow developers to step through the code and identify the source of errors.
# Example of a unit test:
def test_calculate_mean():
assert calculate_mean([1, 2, 3]) == 2
assert calculate_mean([4, 5, 6, 7]) == 5.5
# Example of using a debugger:
def calculate_mean(values):
import pdb; pdb.set_trace()
total = sum(values)
count = len(values)
return total / count
calculate_mean([1, 2, 3])
Follow coding standards and style guides
Coding standards and style guides provide a set of guidelines for formatting, naming, and organizing code. Adhering to these standards can help make the code more readable, maintainable, and reusable. For example, a style guide might specify conventions for indentation, line length, and naming conventions.
# Good example:
def calculate_mean(values):
"""
Calculate the mean of a list of values.
Parameters:
values (list): A list of numerical values.
Returns:
float: The mean of the values.
"""
total = sum(values)
count = len(values)
return total / count
# Bad example:
def calculate_mean(values):
total = sum(values)
count = len(values)
return total / count
Design for maintainability and extensibility
Code that is designed for maintainability and extensibility is easy to update and modify as the needs of the project change. This can save time and effort and can help improve the long-term viability of the code. To design for maintainability and extensibility, developers can use techniques such as modularity, abstraction, and encapsulation, and can follow design patterns that are known to be effective.
# Good example:
class Customer:
def __init__(self, name, address, phone_number):
self.name = name
self.address = address
self.phone_number = phone_number
def update_address(self, new_address):
self.address = new_address
# Bad example:
def update_customer_address(customer, new_address):
customer['address'] = new_address
Continuously integrate and deploy
Continuous integration and deployment practices allow developers to integrate and deploy code changes frequently, reducing the risk of errors and making it easier to deliver new features and updates to users. Continuous integration involves regularly merging code changes into a shared repository and automatically building and testing the code to ensure it is working as expected. Continuous deployment involves automatically deploying code changes to production environments as soon as they are tested and approved.
# Set up a continuous integration pipeline
git push origin master
# Set up a continuous deployment pipeline
git push origin master
By following these best practices, developers can write code that is more maintainable, more readable, and more efficient. This can help to improve the overall quality of the code and make it easier for others to work with and understand.